How to Remove Registration Route from Laravel Auth
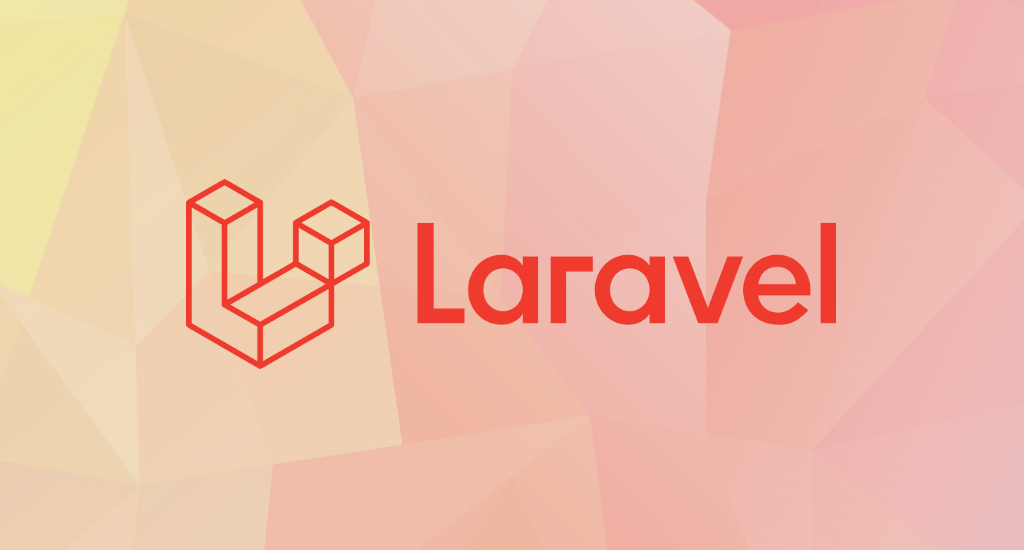
Laravel provides a quick way to scaffold all of the routes and views you need for authentication using one simple command:
$ php artisan make:auth
Usually, this command is used on fresh applications. It will install a layout view, registration and login views, as well as routes for all authentication end-points. A HomeController
will also be generated to handle post-login requests to your application's dashboard.
It will also install several authentication controllers, which are located in the App\Http\Controllers\Auth
namespace.
RegisterController
handles new user registration.LoginController
handles authentication.ForgotPasswordController
handles e-mailing links for resetting passwordsResetPasswordController
contains the logic to reset passwords.
Each of these controllers uses a trait to include their necessary methods. For many applications, you will not need to modify these controllers at all.
But there may be times when you need the authentication part but you don't want people to be able to register for your site. It might be a private back-end that you'll want to create users for manually, or you may have internal tools for inviting new users. Luckily it's pretty easy to strip registration out of default Laravel's scaffold.
Update: If you're using Laravel < 5.7, go on. But if you're using Laravel 5.7 and newer, jump to Laravel 5.7 section on this article.
Remove Route (Laravel < 5.7)
After you called artisan make:auth command, it will add Auth::routes()
on your routes/web.php
file. It's actually calling on Illuminate\Routing\Router::auth()
which you can find it on your vendor/laravel/framework/src/Illuminate/Routing/Router.php
. Let's take a look at how it works under the hood - it literally registers the predefined authentication routes.
/**
* Register the typical authentication routes for an application.
*
* @return void
*/
public function auth()
{
// Authentication Routes...
$this->get('login', 'Auth\LoginController@showLoginForm')->name('login');
$this->post('login', 'Auth\LoginController@login');
$this->post('logout', 'Auth\LoginController@logout')->name('logout');
// Registration Routes...
$this->get('register', 'Auth\RegisterController@showRegistrationForm')->name('register');
$this->post('register', 'Auth\RegisterController@register');
// Password Reset Routes...
$this->get('password/reset', 'Auth\ForgotPasswordController@showLinkRequestForm')->name('password.request');
$this->post('password/email', 'Auth\ForgotPasswordController@sendResetLinkEmail')->name('password.email');
$this->get('password/reset/{token}', 'Auth\ResetPasswordController@showResetForm')->name('password.reset');
$this->post('password/reset', 'Auth\ResetPasswordController@reset');
}
You can see how the routes are broken up into three groups - authentication, registration and password reset. What you need to do is just copy the routes you want to use into you routes/web.php
file and ditch the rest. You'll also need to replace $this->
with Route::
.
After you've replaced $this->
with Route::
and put it on your routes/web.php
, there's no need to call Auth::routes()
again. Remove it from your routes/web.php
file and just leave behind the authentication and password reset routes. Registration will no longer be available in your app and the routes that would have linked to it are no longer present.
If you still confused, here's your routes/web.php
supposed to be:
// Authentication Routes...
Route::get('login', 'Auth\LoginController@showLoginForm')->name('login');
Route::post('login', 'Auth\LoginController@login');
Route::post('logout', 'Auth\LoginController@logout')->name('logout');
// Password Reset Routes...
Route::get('password/reset', 'Auth\ForgotPasswordController@showLinkRequestForm')->name('password.request');
Route::post('password/email', 'Auth\ForgotPasswordController@sendResetLinkEmail')->name('password.email');
Route::get('password/reset/{token}', 'Auth\ResetPasswordController@showResetForm')->name('password.reset');
Route::post('password/reset', 'Auth\ResetPasswordController@reset');
Laravel 5.7 and Newer
This is the updated code on Laravel 5.7. It allows you to simply add options when you call Auth::routes()
.
/**
* Register the typical authentication routes for an application.
*
* @param array $options
* @return void
*/
public function auth(array $options = [])
{
// Authentication Routes...
$this->get('login', 'Auth\LoginController@showLoginForm')->name('login');
$this->post('login', 'Auth\LoginController@login');
$this->post('logout', 'Auth\LoginController@logout')->name('logout');
// Registration Routes...
if ($options['register'] ?? true) {
$this->get('register', 'Auth\RegisterController@showRegistrationForm')->name('register');
$this->post('register', 'Auth\RegisterController@register');
}
// Password Reset Routes...
if ($options['reset'] ?? true) {
$this->resetPassword();
}
// Email Verification Routes...
if ($options['verify'] ?? false) {
$this->emailVerification();
}
}
So if you want to remove registration from Laravel authentication's scaffolding, you may disable it by removing the app/Http/Controllers/Auth/RegisterController.php
and modifying your route declaration: Auth::routes(['register' => false]);
.
Final Words
I hope that you now know how to remove registration from Laravel Authentication Scaffolding. If you run into any issues or have any feedback feel free to drop a comment below.