Laravel Accessors: How to Use and When Do We Use It
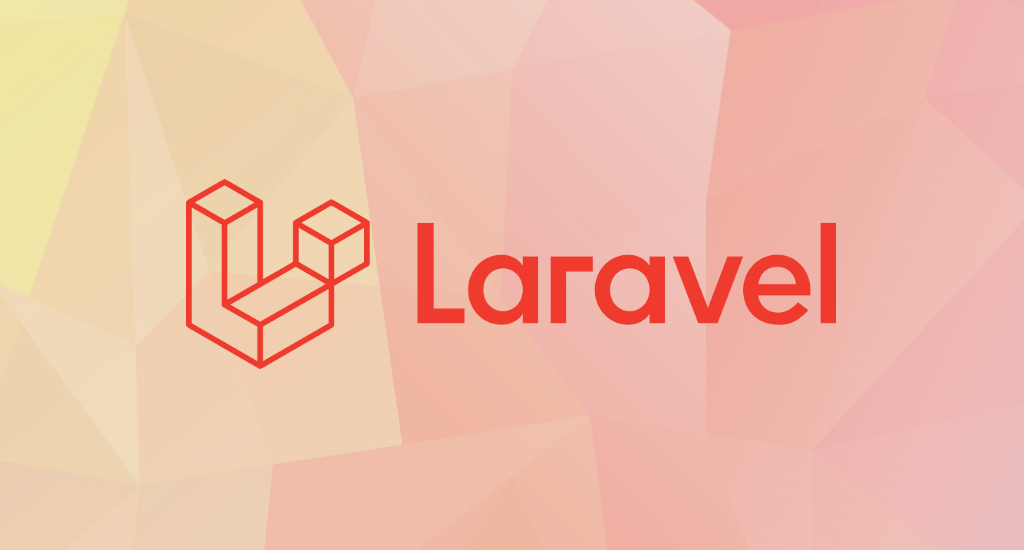
Problem
Eloquent ORM included with Laravel provides a simple ActiveRecord implementation for working with database. Each database table has a corresponding "Model" which is used to interact with that table. Models allow you to query for data in your tables, as well as insert new records into the table.
Many of us stores flag/status field as a number/integer in table's field. For example, let's say we are building an e-commerce website. We know that an order may have some status while processed, like "created", "paid", "shipping", etc. Some of us would store to database as an ENUM field type and the rest would store it as INT/SMALLINT field type. As a developer, when we store status field as an integer we know what status each number refers to. We know that order with status equal to 0 is created, 1 is paid, and so on. We could then show the status in the view using this code:
{{ $order->status }}
The problem is: It will show the status as a number, which off course our visitor/customer wouldn't have a clue about it.
Solution
Luckily enough, the Eloquent ORM has a function to solve this case: Accessors. From Laravel documentation website, it's mentioned that accessors are used to format attributes when you retrieve them from the database. How could we implement this function into our order status?
Step 1
We need to define what are the statuses in our Order model:
protected $readable_statuses = ['Created, 'Paid', 'Shipping', 'Closed'];
Step 2
Then we told this model how to find order status:
public function getReadableStatusAttribute()
{
return $this->readable_statuses[$this->status];
}
Step 3
On our view, we could show the readable status just like we show other order's attribute:
{{ $order->readable_status }}
Done
That's it. We now have a nice readable status from our order dataset.
Final Words
I hope that you now know how to use Laravel Accessor and when do we use it. If you run into any issues or have any feedback feel free to drop a comment below.