Laravel Login with Email or Username in One Field
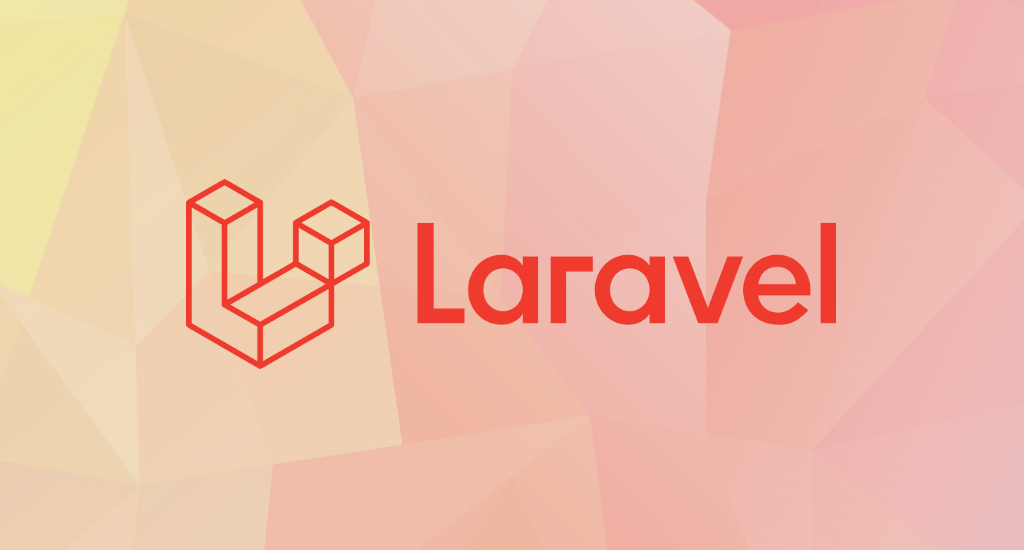
When logging in to a Laravel application, we usually needs to enter email and password. However, there was a client asked to make the login works with either email or username. After digging around, it turns out to be an easy task. How is it possible?
Laravel default authentication allows you to login with an email only but we can also use various other|field like username. Laravel by default only allows one field email for login but we can utilize username with it as well.
Please note that your table should has 'username
' field. If not, you can generate it with slug or something like that. Here's my migration for reference:
// ... previous rows skipped
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('username');
$table->string('email')->unique();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
// ... remaining rows omitted
Modify the app/Http/Auth/LoginController.php
and modify Laravel's default 'login
' method.
public function login(Request $request)
{
$this->validate($request, [
'login' => 'required',
'password' => 'required',
]);
$login_type = filter_var($request->input('login'), FILTER_VALIDATE_EMAIL )
? 'email'
: 'username';
$request->merge([
$login_type => $request->input('login')
]);
if (Auth::attempt($request->only($login_type, 'password'))) {
return redirect()->intended($this->redirectPath());
}
return redirect()->back()
->withInput()
->withErrors([
'login' => 'These credentials do not match our records.',
]);
}
}
I will write a short explanation for each modification made in the Login Controller. We've got added a new $login_type
variable which we will use to keep whether the login
field is email
or username
. This field will only have values email or username. We got the value from filter_var function, checking whether the value being evaluated is a valid email or not.
If the evaluated value is a valid email (fulfilling FILTER_VALIDATE_EMAIL
), we set $login_type
to email
otherwise username
. Then we set this variable with the input login inside the request by using the merge method. Merge method essentially merge a new input into the current request’s array.
Next is test it and see if it works!
Final Words
I hope that you now know how to allow Laravel login with either email or username. If you run into any issues or have any feedback feel free to drop a comment below.