Generating Dummy Data in Laravel Application Using Faker
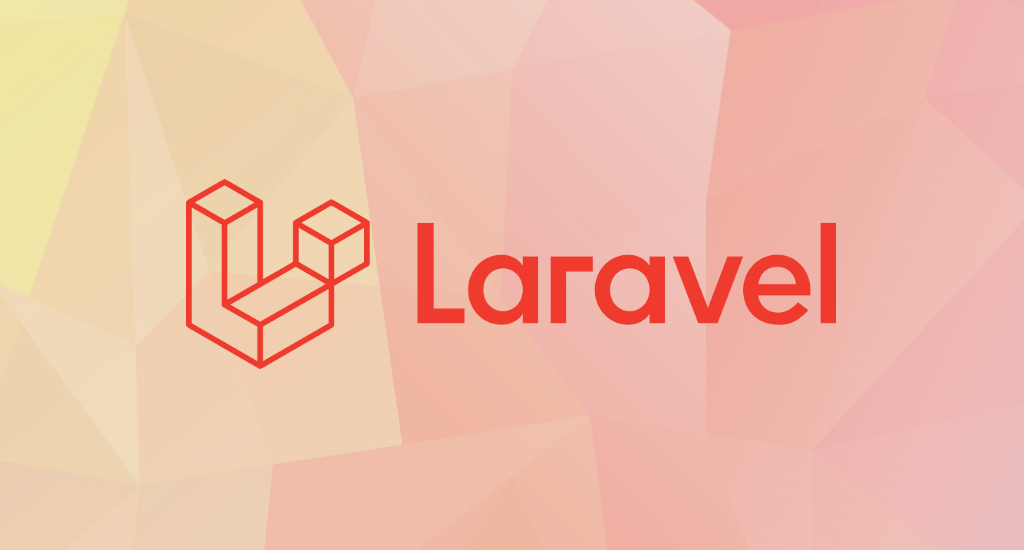
Background
Back in the good old days, I often test PHP applications by accessing it directly from the web browser and input the data in the forms. Today, with the explosion of awesome PHP libraries we can now generate most kinds of data by using code alone. The data can then be directly inserted into the database. This reduces the need to input data directly into the app. In Laravel application, there’s an awesome package for this called Faker - a PHP library that generates fake data for you. Faker is not exactly Laravel package, but perfectly works with this framework as well. Check their github page: https://github.com/fzaninotto/Faker
Also, in a proper development and deployment process, we must test the application everytime we update/change the code/function. Faker could generate fake data, but “real enough” to test how it would look and work.
So, what can Faker do? Basically, fake some data for you. Usually, in Laravel case, it is used within the Seeds. So let’s see real life situation.
Installation
If you already using Laravel, good news – it’s already installed for you in Laravel! But if you're using another framework, you can install Faker by executing the following command. Note that this requires you to have Composer installed.
composer require fzaninotto/faker
Concepts
Here are a few concepts that you need to remember before moving on.
- generators – responsible for generating data.
- providers – the data source for generators. Generators can’t really stand by themselves. Providers really shine on the localization feature of Faker. Real places, phone numbers in countries can be generated by Faker through the use of providers.
- formatters – these are the properties that you can access from a specific generator. Examples include name, city, address, and phoneNumber.
Usage
Let’s take an example from the official Laravel documentation on Seeding. As the framework comes with users table migration, we will use it for our example. Here’s how seed file (database/seeds/DatabaseSeeder.php
) looks:
<?php
use Illuminate\Database\Seeder;
use Illuminate\Database\Eloquent\Model;
class DatabaseSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
DB::table('users')->insert([
'name' => str_random(10),
'email' => str_random(10).'@gmail.com',
'password' => bcrypt('secret'),
]);
}
}
Based on our example above, we will rewrite the seed using Faker and generating 10 users instead of 1:
<?php
use DB;
use Illuminate\Database\Seeder;
use Illuminate\Database\Eloquent\Model;
use Faker\Factory as Faker;
class DatabaseSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
$faker = Faker::create();
foreach (range(1,10) as $index) {
DB::table('users')->insert([
'name' => $faker->name,
'email' => $faker->email,
'password' => bcrypt('secret'),
]);
}
}
}
That’s it – $faker->name
will generate a random person name, and $faker->email
– a random email. After we run artisan db:seed
, results would look something like this:
Most Common Used Formatters
Here are the formatters that I commonly use in my projects.
<?php
//person
$faker->name;
$faker->firstName('female');
$faker->lastName;
//address
$faker->address;
$faker->streetName;
$faker->streetAddress;
$faker->postCode;
$faker->address;
$faker->country;
//company
$faker->company;
//date and time
$faker->year;
$faker->month; //number representation of a month
$faker->monthName;
$faker->timezone; //valid php timezone (http://php.net/manual/en/timezones.php)
$faker->time; //string time
$faker->dateTime; //datetime object
$faker->unixTime; //unix timestamp
//internet
$faker->email;
$faker->userName;
$faker->password;
Localization
Since Faker is an open-source project that anyone can contribute to, lots of localized providers has already been added. You can take advantage of this by passing in the locale when you create a new Faker instance. Please check their available localization lists: https://github.com/fzaninotto/Faker/tree/master/src/Faker/Provider
For example, if you live in the Indonesia:
$faker = Faker::create('id_ID');
Future Date
To generate future date,You can pass strtotime
string conditions to $faker->dateTimeBetween()
. You can see http://php.net/manual/en/function.strtotime.php for a full list of string usages and combinations.
//generate datetime between today and next 2 years
$faker->dateTimeBetween('+0 days', '+2 years')
//generate datetime between next week and next month
$faker->dateTimeBetween('+1 week', '+1 month')
// ... etc
Conclusion
Say goodbye to those good old days. Whether we develop with or without Laravel, we can easily testing and generate dummy data using this Faker package.