Logging Last Login Information Using Laravel Events
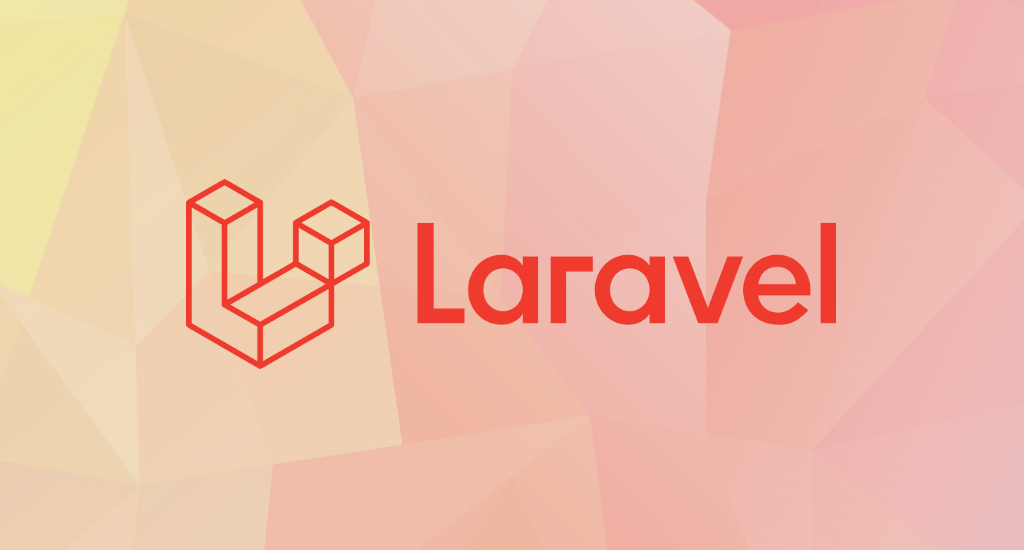
Laravel's events provides a simple observer implementation, allowing you to subscribe and listen for various events that occur in your application. Events serve as a great way to decouple various aspects of your application, since a single event can have multiple listeners that do not depend on each other. So, we'll use Laravel Events to log each user's login information.
Registering Events
Edit the EventServiceProvider
included in your Laravel application to register the event listener.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Event;
use Illuminate\Foundation\Support\Providers\EventServiceProvider as ServiceProvider;
class EventServiceProvider extends ServiceProvider
{
/**
* The event listener mappings for the application.
*
* @var array
*/
protected $listen = [
'Illuminate\Auth\Events\Login' => [
'App\Listeners\LogSuccessfulLogin',
],
];
/**
* Register any events for your application.
*
* @return void
*/
public function boot()
{
parent::boot();
//
}
}
Use the artisan event:generate
command to generate the LogSuccessfulLogin
listener.
Edit the LogSuccessfulLogin
listener to add the event handling logic.
<?php
namespace App\Listeners;
use Illuminate\Http\Request;
use Illuminate\Auth\Events\Login;
class LogSuccessfulLogin
{
/**
* Create the event listener.
*
* @param Request $request
* @return void
*/
public function __construct(Request $request)
{
$this->request = $request;
}
/**
* Handle the event.
*
* @param Login $event
* @return void
*/
public function handle(Login $event)
{
$user = $event->user;
$user->last_login_at = date('Y-m-d H:i:s');
$user->last_login_ip = $this->request->ip();
$user->save();
}
}
Update table structure
To be able to store the login information to database, we need to modify users table.
$ php artisan make:migration add_last_login_info_to_users_table --table=users
Add the last_login_at
and the last_login_ip
columns.
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class AddLastLoginInfoToUsersTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->timestamp('last_login_at')->nullable()->after('remember_token');
$table->string('last_login_ip')->nullable()->after('last_login_at');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn(['last_login_at']);
$table->dropColumn(['last_login_ip']);
});
}
}
Last step is do the migration:
$ php artisan migrate
Update May 11, 2019
In most cases, above code is enough. But how if we don't want to update "updated_at" field? It's very easy to solve with Query Builder. Just update the listener LogSuccessfulLogin
with code below:
<?php
namespace App\Listeners;
use DB;
use Illuminate\Http\Request;
use Illuminate\Auth\Events\Login;
class LogSuccessfulLogin
{
/**
* Create the event listener.
*
* @param Request $request
* @return void
*/
public function __construct(Request $request)
{
$this->request = $request;
}
/**
* Handle the event.
*
* @param Login $event
* @return void
*/
public function handle(Login $event)
{
$user = $event->user;
DB::table('users')
->where('id', $user->id)
->update([
'last_login_at' => date('Y-m-d H:i:s'),
'last_login_ip' => $this->request->ip(),
]);
}
}
Final Words
I hope that you now know how to logging last login information using Laravel Events. If you run into any issues or have any feedback feel free to drop a comment below.
Add columns to users model $fillable
I am genuinely delighted to glance at this webpage posts which consists of lots of helpful information, thanks for providing these information.
works like a charm, thanks, just one question is it possible for updated at to not update too?
Great question. I've updated my post with explanation.