How to Apply Default Ordering on Laravel Eloquent Model
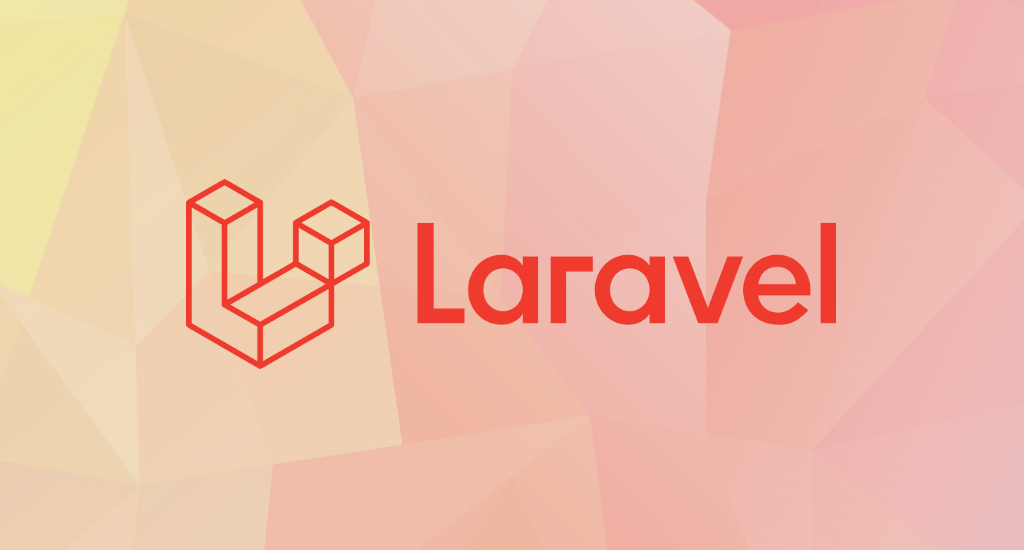
Most of the time when we want to display a dropdown or list all records on database, we need to order the column in same condition throughout the application. Calling orderBy
everytime we need to display the data could be avoided using Global Scope.
For example, we will use country
data. Let's say we want to always show countries ordered by name attribute in ascending direction. This could be easily implemented with modification on Country
model below:
namespace App;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Builder;
class Country extends Model
{
protected static function boot()
{
parent::boot();
// Order by name ASC
static::addGlobalScope('order', function (Builder $builder) {
$builder->orderBy('name', 'asc');
});
}
// ... rest of lines omitted
Notice what changed from usual model definition on code snippet above. We add this line:
use Illuminate\Database\Eloquent\Builder;
and override boot
function. This function calls addGlobalScope
method by using anonymous function as a parameter, where we actually tell the system what condition to add to all operations with Query Builder.
After we save the file and call Country::all()
, it will actually execute this query:
SELECT * FROM `countries` ORDER BY `name` ASC
Final Words
Well, that's it. I hope that you now know how to solve apply default ordering on Laravel Eloquent Model. If you run into any issues or have any feedback feel free to drop a comment below.