Laravel 5.7 Guzzle HTTP Client Request Example
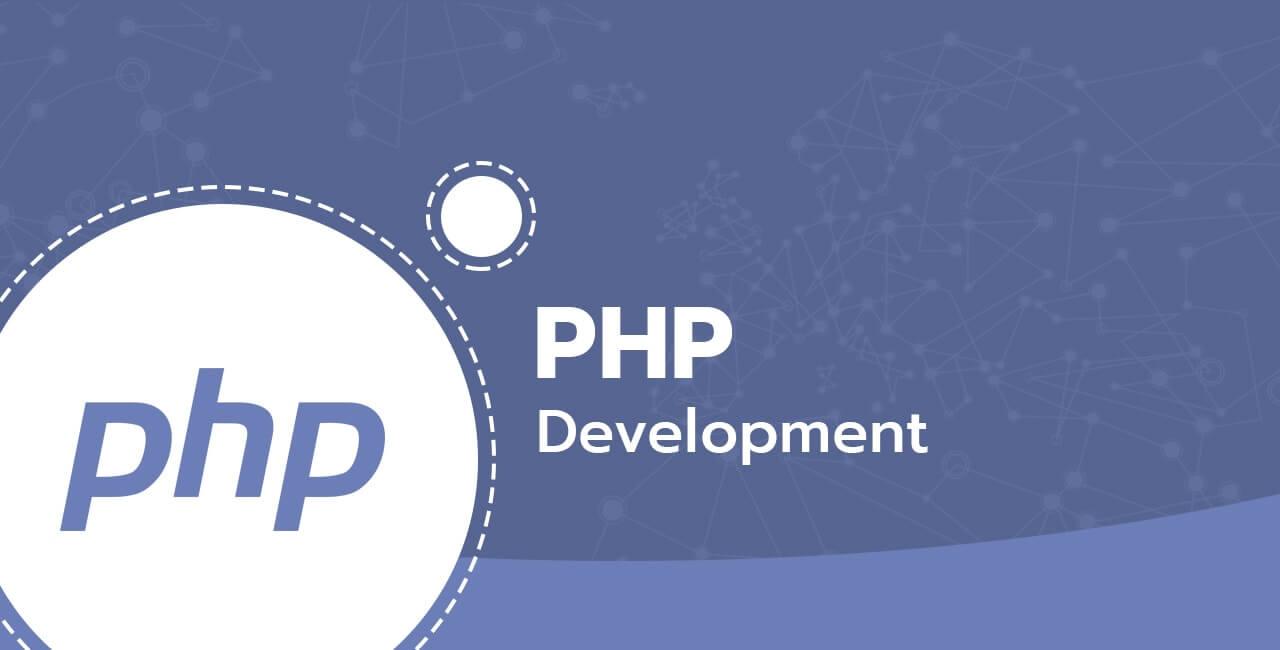
Web application development cannot be separated with communicating with 3rd party API. I'll write an example of how using guzzle package on Laravel framework. Although I uses Laravel 5.7, the code mentioned here should be similar with any Laravel 5.* version.
What is Guzzle
Guzzle is a PHP HTTP client that simplify HTTP requests manipulation. This make our life as as web developer easier to integrate with REST APIs. Guzzle provides a simple interface for building query strings, POST requests, streaming large uploads, streaming large downloads, using HTTP cookies, uploading JSON data, etc. Guzzle can also send both synchronous and asynchronous requests using the same interface.
Note:
Guzzle no longer requires cURL in order to send HTTP requests. Guzzle will use the PHP stream wrapper to send HTTP requests if cURL is not installed. Alternatively, you can provide your own HTTP handler used to send requests.
Laravel Integration
Installation
It's a little strange if you're not familiar with composer yet. If that's the case, you might want to read about composer on their website.Or, if you want to use Guzzle outside Laravel project, this post might help you.
On your root laravel project directory, type this command:
$ composer require guzzlehttp/guzzle
Creating a Client
On your controller, include the Guzzle package:
use GuzzleHttp\Client;
Then instantiate a new Client object on your function
public function index()
{
// Create client
$client = new Client([
// Example
'base_uri' => 'https://www.google.com',
]);
}
Note:
Clients are immutable in Guzzle 6, which means that you cannot change the defaults used by a client after it's created.
Sending Synchronous Request
There are two available methods:
// Send request and collect response
$response = $client->get('https://www.google.com');
and
use GuzzleHttp\Psr7\Request;
// .. code omitted
$request = new Request('GET', 'https://www.google.com');
$response = $client->send($request, ['timeout' => 2]);
Another Request Type
There are another request type available:
- Async request - Useful to handle request with long-time response possibility.
- Concurrent request - Simple usage: scraping.
I'll write about them on another post.
Processing Response
After the request is sent, we would expect some response from the server. Here's how we get the response and print it on web browser.
// .. code omitted
// Processing response
$body = $response->getBody();
$html_string = (string) $body;
// Dump on browser
dd($html_string);
When you open your application route on web browser, you'd expect something like this:
Full Code
Here's my full code:
public function index()
{
// Create client
$client = new Client([
// Example
'base_uri' => 'https://www.google.com',
]);
// Send request and collect response
$response = $client->get('https://www.google.com');
// Processing response
$body = $response->getBody();
$html_string = (string) $body;
// Dump on browser
dd($html_string);
}
Final Words
I hope that you now know how to use Guzzle on Laravel 5.7. If you run into any issues or have any feedback feel free to drop a comment below.