How to Validate File Upload in Laravel 5 with MIME Types or File Extensions
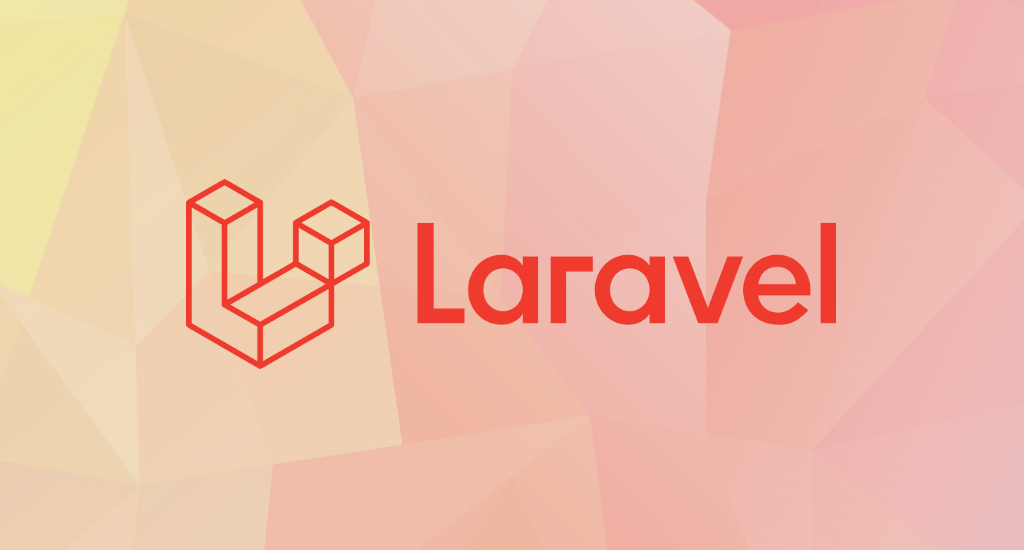
Most of the times when it comes to web application development, we as a developer need to either check or validate uploaded file from user. This routine is a great practice, to prevent user from uploading malicious file. Luckily on Laravel, this could be done in easy way.
Note: This guide will only apply to Laravel > 5
There are two approach to validate uploaded file: by checking MIME types and by file extension.
File extension
Suppose on your HTML you have file input with avatar
name. Logically, this avatar
file input would only accept valid image formats. So, on your controller you could add this rule to validate uploaded file extensions.
$validator = Validator::make($request->all(), [
'avatar' => 'mimes:jpeg,png,bmp,gif,svg',
]);
Image upload
Special case for image, you could user another validation method: image. So, instead of defining mime types like example above, you could use this rule:
$validator = Validator::make($request->all(), [
'avatar' => 'image',
]);
The problem with the MIME validation type is that it does not actually validate against the MIME type, but rather the extension of the file that is assumed from the mime type.
MIME types
Starting from Laravel 5.2, you could validate file upload against full MIME types, text/plain
for example. Consider you want to allow your user to upload introductory video about themself. In that case, we define valid MIME types for videos. Here's what we'll have on the controller validation rule:
$validator = Validator::make($request->all(), [
'video' => 'mimetypes:video/avi,video/mpeg,video/quicktime'
]);
If you would like to check full listing of MIME types and their corresponding extensions, it depends on your web server.
Nginx web server
To check supported MIME types on Nginx web server, issue following command on your shell:
$ cat /etc/nginx/mime.types
Apache web server
For Apache web server, you could check supported MIME types here.
Final Words
I hope that you now know how to validate file upload in Laravel 5 with MIME types or file extensions. If you run into any issues or have any feedback feel free to drop a comment below.
It is better to validate on the basis of file extension, which can easily be done using following command
$allowedfileExtension=['pdf','jpg','png','docx'];
This code will go into the controller of Laravel file upload form.